In This Tutorial, We are going to learn how we can enter or type in the text box using the send keys.
and also we will learn another method to enter the text in the textbox without using the send keys.
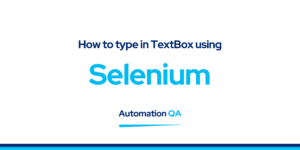
Method 1: How to Enter Text in Text Box Using Send Keys
Sendkeys Mehotd: In Selenium sendkeys() Method is used to type or enter the text into the textbox.
Syntax : driver.findElement(By.id(“text-bx”)).sendKeys(“Automation QA”)
Selenium Code:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class InputTxt{
public static void main(String[] args) {
System.setProperty(“webdriver.chrome.driver”,
“C:\Users\ghs6kor\Desktop\Java\chromedriver.exe”);
WebDriver driver = new ChromeDriver();
String url = “Enter URL HERE”;
driver.get(url);
driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS);
// identify element and input text inside it
WebElement l =driver.findElement(By.className(“gsc-input”));
l.sendKeys(“Selenium Automation”);
driver.quit();
}
}
How to Type in Text Box Without using Sendkey in Selenium using Java
Method 2:How to Enter Text in Text Box Without Sendkeys Method
To type in the text box without using the send keys method we use JavaScript Executor. Selenium executes JavaScript commands with the help of the executeScript method.
Syntax :
JavascriptExecutor j = (JavascriptExecutor)
driver; j.executeScript (“document.getElementById(‘gsc-i-id1’).value=’Selenium'”);
Selenium Code:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.JavascriptExecutor;
public class JsEnterText{
public static void main(String[] args) {
System.setProperty(“webdriver.gecko.driver”,
“C:\Users\ghs6kor\Desktop\Java\geckodriver.exe”);
WebDriver driver = new FirefoxDriver();
//implicit wait
driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS);
//URL launch
driver.get(“Enter Your URL”);
// JavaScript Executor to enter text
JavascriptExecutor j = (JavascriptExecutor)driver;
j.executeScript (“document.getElementById(‘gsc-i-id1’).value=’Selenium'”);
WebElement l = driver.findElement(By.id(“gsc-i-id1”));
String s = l.getAttribute(“value”);
System.out.println(“Value entered is: “ + s);
driver.quit();
}
}
Also Read:
How to Verify Page Title in Selenium